Normally Laravel has authentication including email verification built in. Since Laravel 5.7 I believe. But sometimes you need it added as you have a Laravel application that predates all this.
Authentication
Basisc authentication we set up using
php artisan ui vue --auth
This command generated all the needed routes, view, controller and middleware.
New Migration
So that means you need an extra migration to add the email_verified_at column to the Users table
php artisan make:migration add_email_verified_at_to_users
The newly created migration has to be tweaked some to add the new column and should look like this
class AddEmailVerifiedAtToUsers extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::table('users', function (Blueprint $table) { $table->timestamp('email_verified_at')->nullable(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::table('users', function (Blueprint $table) { $table->dropColumn(['email_verified_at']); }); } }
Then you can run the migration as per usual
php artisan migrate
That should add the missing column email_verified_at to the table users.
Note
If you live or production server had a more recent installation based upon your code base you may need to check if the column email_verified_at already exists or not otherwise a deployment from Forge or elsewhere will fail
if (!Schema::hasColumn('users', 'email_verified_at')) { $table->timestamp('email_verified_at'); }
Tip from Laravel Daily
User Model Update
It also means you need to update and add MustVerifyEmail implementation to app/User.php
Class User extends Authenticatable implements MustVerifyEmail
Routes
Then need to update the auth routes to use the email verification using:
Auth::routes(['verify' => true]);
instead of
Auth::routes();
Now, you may also have basic routes protected with basic Laravel authentication. These you may want to update to check if email was verified as well.
Local Email Setup
You may need to change your email settings to do local registration tests. You will in that case see an error like
Swift_TransportException Connection could not be established with host localhost :stream_socket_client(): unable to connect to localhost:1025 (Connection refused)
We use MailTrap and the free account only allows one project or mail box. But it works so we re-use
MAIL_DRIVER=smtp MAIL_HOST=smtp.mailtrap.io MAIL_PORT=2525 MAIL_USERNAME=username MAIL_PASSWORD=password MAIL_ENCRYPTION=null
Do not forget a
php artisan config:clear
Registration Trial Run
Now let’s see if things work now shall we? Do a registration on your app. On registration you should now see this as an email (different business details of course):
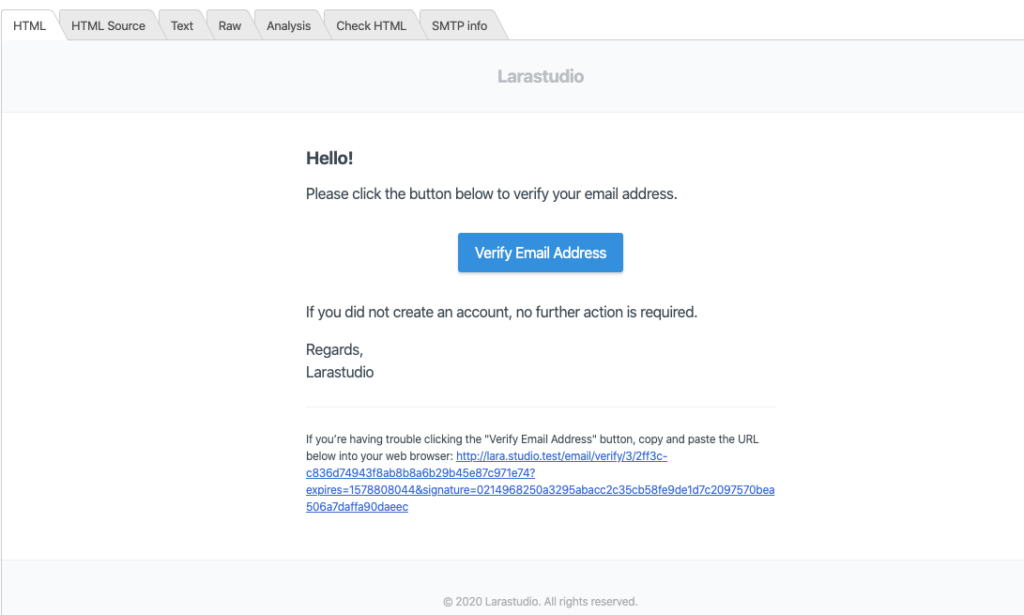
Routes with verification
One issue we had was that it initially still showed that we were logged in and that should not happen of course. This was because we did not add the verified to the routes in question yet.
/* |-------------------------------------------------------------------------- | Dashboard Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your Dashboard. Currently | we only have a basic set up here. | */ Route::middleware([ 'auth', 'verified' ])->group(function () { Route::get('/dashboard', 'DashboardController@index')->name('dashboard'); }); /* |-------------------------------------------------------------------------- | Verification Routes Activation |-------------------------------------------------------------------------- | | Verification routes to work with email verification. | https://github.com/laravel/framework/blob/6.x/src/Illuminate/Routing/Router.php#L1205 | */ Auth::routes(['verify' => true]); /* | ------------------------------------------------------------------------- | Custom Verification Routes as Option | ------------------------------------------------------------------------- | | This will add custom routes like email / verify and email/resend | to our application. | https://stackoverflow.com/a/52576695 | */ // Auth::routes(); // Route::get('email/verify', 'Auth\VerificationController@show')->name('verification.notice'); // Route::get('email/verify/{id}', 'Auth\VerificationController@verify')->name('verification.verify'); // Route::get('email/resend', 'Auth\VerificationController@resend')->name('verification.resend');
Redirect Issues
Other issue was that we hit a 404 locally clicking on the verification button which led to http://lara.studio.test/dasboard . The email was verified however. That was a simple typo issue.